Angular, the fifth most popular web framework with 20.39% popularity as per Statista, has come up with a new & exciting version 15. The angular v15 is live and has been rolled out for the masses.
It is a significant shift for tech enthusiasts, primarily JavaScript & TypeScript programmers and developers. The new release will make remarkable contributions to the digital development world, as there are tons of Angular-based applications in active use.
So without any hither and thither, let’s dive into the details of what’s new with Angular 15
#1. Experience Standalone Components API
The concept of the standalone components API was initially made available in Angular 14. Its objective is to create Angular applications without wasting time defining the NgModules. With Angular 15, the standalone components API is now finally successful, as it has higher performance than its predecessor.
Notably, the standalone component is compatible with HttpClient, Angular Elements, and many others. Coders can also leverage the feature for bootstrapping an application in a solo component.
The following example illustrates how to leverage the standalone components API:
import {bootstrapApplication} from '@angular/platform-browser';
import {ImageGridComponent} from'./image-grid';
@Component({
standalone: true,
selector: 'photo-gallery',
imports: [ImageGridComponent],
template: `
… <image-grid [images]="imageList"></image-grid>
`,
})
export class PhotoGalleryComponent {
// component logic
}
bootstrapApplication(PhotoGalleryComponent);
The imports function now lets Angular developers leverage reference standalone directives & pipes.
The Angular v15 also lets a developer preview and mark components, directives, and pipes as “standalone: true”. Thus, it successfully eliminates the need to declare components into NgModule. Moreover, developers can directly import NgModule into the standalone component using the code: [module_name].
#2. Improved and more efficient use of standalone api
The new release has a router standalone API. It lets developers build the multi-route application. The following example shows how:
export const appRoutes: Routes = [{
path: 'lazy',
loadChildren: () => import('./lazy/lazy.routes')
.then(routes => routes.lazyRoutes)
}];
You can declare the lazyRoutes in the following manner:
import {Routes} from '@angular/router';
import {LazyComponent} from './lazy.component';
export const lazyRoutes: Routes = [{path: '', component: LazyComponent}];
The new release also lets you register the appRoutes via the bootstrapApplication method. One can also call it via the ProvideRouter API, which is tree-shakable!
bootstrapApplication(AppComponent, {
providers: [
provideRouter(appRoutes)
]
});
An essential aspect of Angular 15 is that the developers can eliminate unused features and reduce the code size by up to 11%.
#3. Directive Composition API
Code reusability is a significant factor when looking for faster development cycles and a faster time-to-market application. The Angular 15 offers top-notch reusability of directives. It comes with the introduction of Directive Composition API, which plays a pivotal role in code reusability.
For example, the developers can boost host elements with directives and leverage code reuse strategy with the assistance of a compiler. Along with the standalone directives, programmers can leverage the Directive Composition API
@Component({
selector: 'mat-menu',
hostDirectives: [HasColor, {
directive: CdkMenu,
inputs: ['cdkMenuDisabled: disabled'],
outputs: ['cdkMenuClosed: closed']
}]
})
class MatMenu {}
The MatMenu is now available and effective via two hostDirectives, i.e., HasColor and CdkMenu. Moreover, with this feature, MatMenu lets users have hands on all the properties from these two directives.
You May Find This Interesting : In-Depth Guide To Angular Performance Tuning
#4. Get Your Hands on Stable “NgOptimizedImage” Image Directive
Image loading time plays a vital role in the speed performance of any web application. The Angular framework was introduced with the NgOptimizedImage directive in the older versions to offer minimum image loading time. However, it was far from perfection. With Angular15, the directive is much more stable and is finally a reliable image-loading tool.
Have a look at the experiment done by Land’s End. Using the NgOptimizedImage directive an application has noticed an improvement as impressive as a 75% decrement in the LCP (Largest Contentful Paint) image loading time.
Along with the previously offered features, it also comes with the following:
- Automatic srcset Generation: It deals with the automated generation of srcset, responsible for uploading an appropriate image size under the request, thus reducing the image loading time.
- Fill Mode [experimental]: It is responsible for removing the declaration of image dimensions and instilling an image in the original container. The method is efficient for handling images whose dimensions are not specific or when the developers migrate the CSS background image to leverage image directive and thus is effective in managing inappropriate as well as any appropriately sized image.
You can use the standalone NgOptimizedImage directive in your Angular component or NgModule.
import { NgOptimizedImage } from '@angular/common';
// Include it into the necessary NgModule
@NgModule({
imports: [NgOptimizedImage],
})
class AppModule {}
// ... or a standalone Component
@Component({
standalone: true
imports: [NgOptimizedImage],
})
class MyStandaloneComponent {}
Note: For using the Angular image directive within a component, you must replace the image src attribute with ngSrc. Also, the concerned Angular team needs to mark the priority attribute, which is ideal for optimizing the speed of the LCP images.
#5. Trim Boilerplate in Guards
We will discuss this aspect with the help of an example. Or the sake of clarity, let’s analyze using guard whether the user has logged in:
@Injectable({ providedIn: 'root' })
export class MyGuardWithDependency implements CanActivate {
constructor(private loginService: LoginService) {}
canActivate() {
return this.loginService.isLoggedIn();
}
}
const route = {
path: 'somePath',
canActivate: [MyGuardWithDependency]
};
In the code mentioned above, the LoginService contains vital logic. In contrast, the guard is responsible for only one trigger being invoked, which is LoggedIn (); despite a clear structure, there is an unnecessary or overuse of boilerplate coding, which developers can eliminate effectively.
With Functional Router Guards, it is now possible. For example, the above code can be replaced by the following code.
const route = {
path: 'admin',
canActivate: [() => inject(LoginService).isLoggedIn()]
};
Moreover, Functional Guards are compostable, like cherries on the top of the cake. It lets you develop a Factor-like function, manage a given configuration, and return a function per the programmer’s need.
#6. Neat Stack Traces
Now programmers can count upon clean and straightforward stack traces for simplified application debugging. Earlier the framework gave the coding team only single-liner texts, making managing errors difficult. For example, look at the snippet of the error indication available with the earlier version of the angular framework.
ERROR Error: Uncaught (in promise): Error
Error
at app.component.ts:18:11
at Generator.next (<anonymous>)
at asyncGeneratorStep (asyncToGenerator.js:3:1)
at _next (asyncToGenerator.js:25:1)
at _ZoneDelegate.invoke (zone.js:372:26)
at Object.onInvoke (core.mjs:26378:33)
at _ZoneDelegate.invoke (zone.js:371:52)
at Zone.run (zone.js:134:43)
at zone.js:1275:36
at _ZoneDelegate.invokeTask (zone.js:406:31)
at resolvePromise (zone.js:1211:31)
at zone.js:1118:17
at zone.js:1134:33
There were mainly two issues with this method of error declaration:
- Error messages were from the third-party dependencies, and
- Zero data about the user-facing the errors
In contrast, the new Angular web development framework will offer the following:
ERROR Error: Uncaught (in promise): Error
Error
at app.component.ts:18:11
at fetch (async)
at (anonymous) (app.component.ts:4)
at request (app.component.ts:4)
at (anonymous) (app.component.ts:17)
at submit (app.component.ts:15)
at AppComponent_click_3_listener (app.component.html:4)
From the above example, you can view the error message with detailed insights into the path of the encountered error. This saves time in resolving the issue.
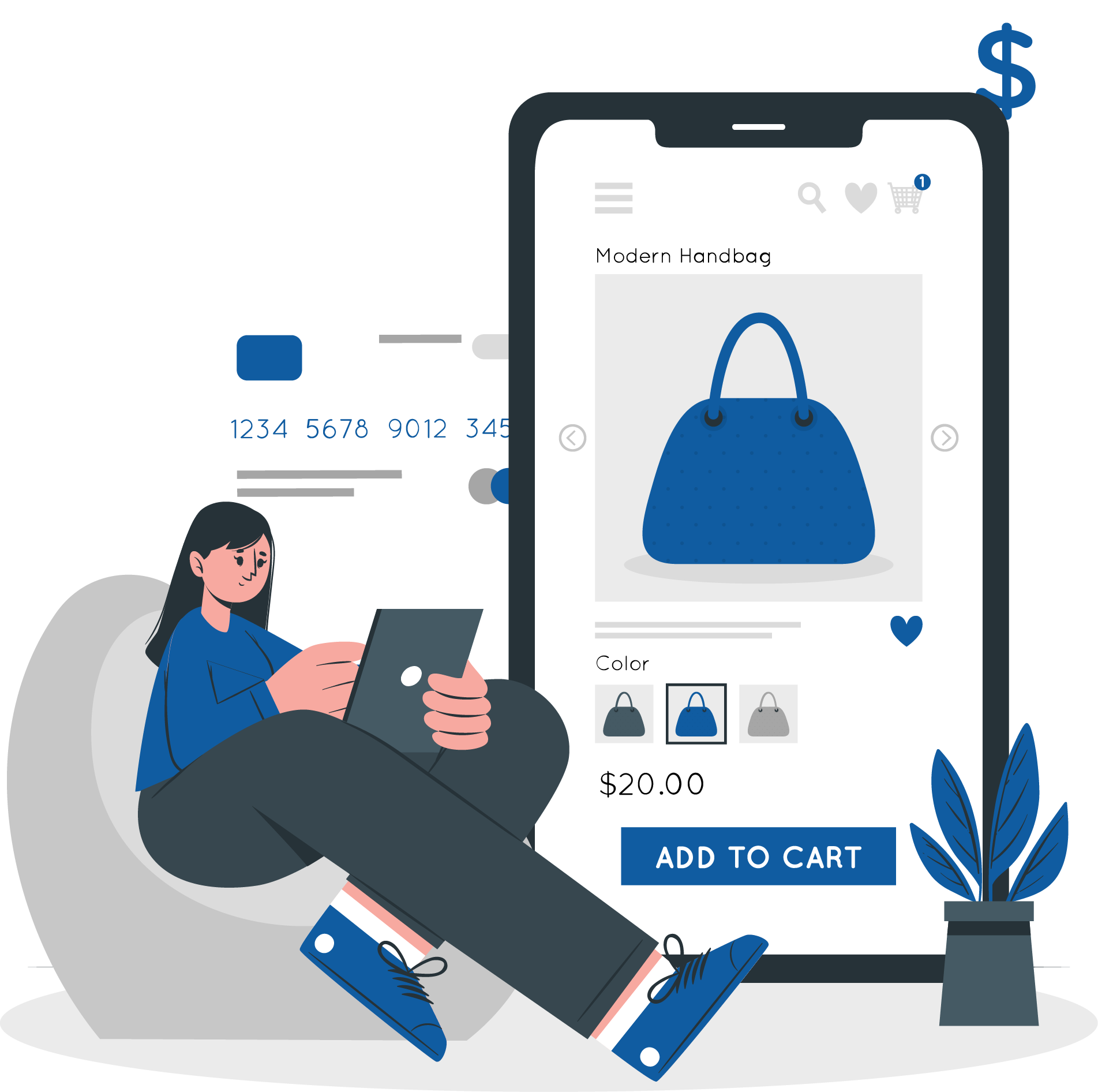
#7. Welcome Stable MDC – Based Components
The upgrade team has done a praise-worthy job refactoring the component based on the Angular Material Design Components for the web applications. The upgraded components will give users greater accessibility and are coherent with the Angular Material Design Specifications.
A significant refactoring section is visible in the DOM and CSS parts. With this new take on responsiveness, developers will experience a few styles in the old Angular applications requiring adjustments. It will be prominent in the case of CSS overriding internal elements of the migrated components.
Moreover, the new version has deprecated many components, which can be recovered with the “legacy” import. The following is an example of retrieving old mat-button implementation:
import {MatLegacyButtonModule} from '@angular/material/legacy-button';
#8. Component Dev Kit Listbox
The CDK Listbox assists in designing and developing UI components. The new release comes with a CDK Listbox that facilitates customization of the Listbox interactions drawn up by the WAI-ARIA Listbox pattern per the project’s needs.
You get hands-on behavioral interactions, which include the following:
- Keyboard Interactions
- Bidi Layout Support
- Focus Management.
Each directive has an associated ARIA role with the concerned host elements.
#9. Leverage Extended esbuild Support
The Angular 15 comes with innovative yet experimental support for the ng build, including the following
- Watch Support,
- Sass
- SVG Template
- File Replacement.
The command for leveraging the upgrade on the angular.json builder is as follows:
"builder": "@angular-devkit/build-angular:browser-esbuild"
#10. Few Significant Enhancements with Angular v15
Following are some other significant new enhancements that are bound to play a pivotal role in enhancing the UX of the developed application.
Lazy Loading Improvement
The router can now auto-unwrap the default exports for lazy loading the images. The result is reduced boilerplates as the router functionalities have been significantly simplified. In simple words, the router can now directly look for the default export, which facilitates directly importing the lazy-file into the code when successful.
Automatic Imports
The new Angular v15 brings greater confidence for the coders as they can make the most out of the language service. It automatically import components and their fixes in the template. It is applicable for the components otherwise not utilized in the standalone component or a NgModule.
Improved Angular Components
Experience a range of accessibility enhancements, including the following:
# Effective contrast ratios
# Expanded touch target sizes
# Refined ARIA semantics
# Significant API customization for a personalized experience.
Wrapping Up
That was all about the new features available with Angularv15. If your business is running on the Angular framework, it’s time to update to the latest version. You cannot afford to miss the new and exciting features that enhance the speed and other aspects of the application’s performance.
Hire Angular developer to upgrade your business application to the latest version of the frontend framework.
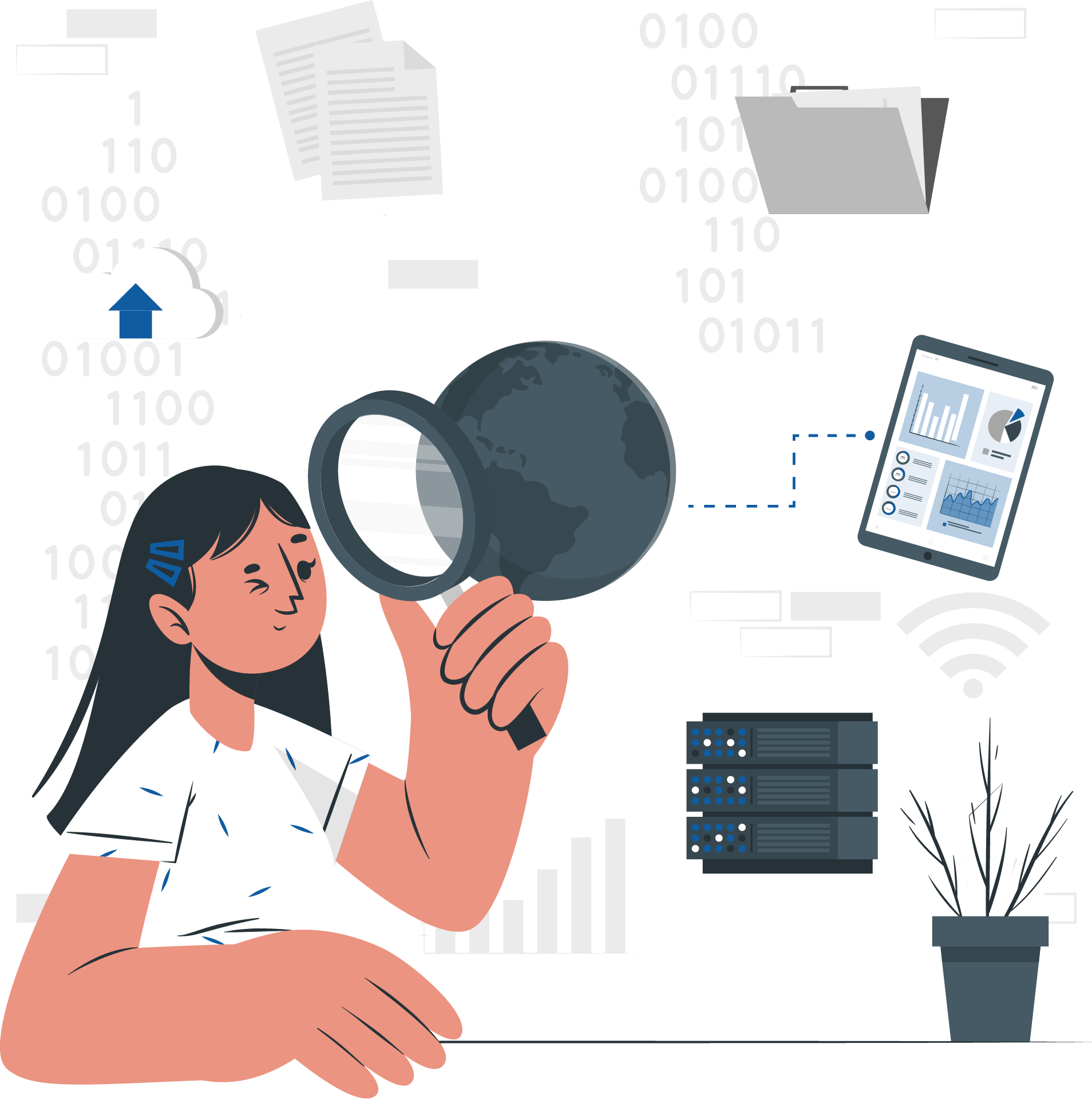
2500+
Project Launched
20+
Years of Experience
250+
Skilled Professionals
60+
Days of Free Support